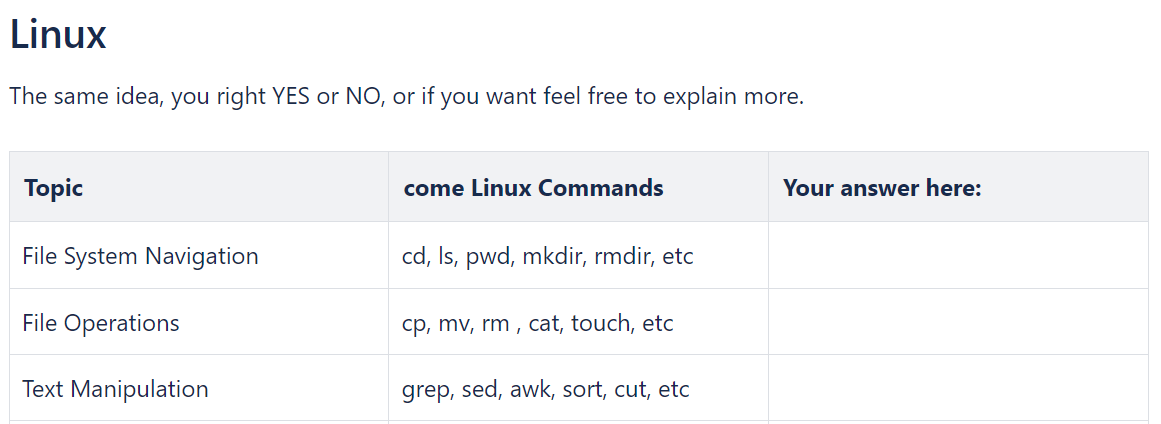
Quotas and why do you need them?
API quotas are restrictions placed on the maximum number of requests that can be made to an API in a specific time period, typically a day or a month.
Some restrictions have been put in place to ensure that the API is used fairly by all users and to prevent server overload. API quotas can be determined by a number of criteria, such as the number of requests made per user, per IP address, or per API key.
- Managing server load: APIs are hosted on servers that have a finite amount of resources. If too many API calls are made in a short period of time, it can cause the server to become overloaded and result in decreased performance or even downtime. By setting quotas, API providers can limit the number of requests and spread them out over time to manage server load and ensure the API remains available.
- Preventing abuse: API quotas can prevent abusive or malicious use of the API. For example, if an application or user makes an excessive number of requests in a short period of time, it may indicate an attempt to overload the server, steal data, or conduct a denial-of-service attack. Quotas can help prevent such abuse and protect the API and its users.
- Managing costs: API providers may have to pay for the resources used to support API requests. By setting quotas, they can manage costs and ensure that they are not charged for excessive usage by individual applications or users.
There are quotas for some requests, such as read versus write requests, in some APIs. If a user makes more API calls than allowed, they can get an error notice or be briefly prevented from doing so. Monitoring consumption is crucial to avoiding exceeding API quotas.
Exceeding API quotas can have several consequences, including:
- Increased latency: When an application or user exceeds their API quota, subsequent requests may be delayed or blocked until the quota resets. This can increase the response time of the API and lead to poor user experience.
- Error messages: If an application or user exceeds their API quota, they may receive error messages or status codes indicating that their request has been rejected or limited.
- Service interruption: In some cases, exceeding API quotas can lead to service interruption, where the API provider may need to temporarily suspend the API or limit access to certain users or applications.
To ensure that API quotas aren’t set too low, API providers should consider several factors, such as usage patterns. API providers should analyse usage patterns and historical data to determine the typical number of requests made by users and applications. This can help them set quotas that are sufficient to meet demand while avoiding overage. API providers should also consider the availability of their resources, such as server capacity and bandwidth. To avoid service interruptions or degradation, quotas should be set at a level that can be supported by the available resources.
API providers should regularly monitor usage patterns and adjust quotas as necessary to ensure that they remain appropriate and effective. By taking a data-driven and user-focused approach, API providers can set quotas that strike the right balance between availability, performance, and user satisfaction.
There are too many requests
The ability to design, deploy, and manage APIs at scale is one of AWS’s most crucial services, and it is called API Gateway. Nonetheless, controlling the use of APIs can be difficult, particularly when dealing with a large number of users and erratic traffic patterns.
API Gateway Usage Plans can help with that…
A helpful tool for controlling API access and spending on Amazon is usage plans. In order to make sure that users are appropriately charged for the resources they use, they give customers the ability to set up consumption tiers and rate restrictions for their APIs. We will go over the advantages of usage plans and their operation in this article.
How do API Gateway Usage Plans work?
Usage plans provide a way to control and monitor access to APIs deployed in API Gateway. They allow API owners to set throttling and quota limits for individual API keys, which can be associated with different groups of users or applications.
Photo by Jon Moore on Unsplash
Here’s how usage plans work in more detail:
- The usage plan that an API owner provides, creates the throttling and quota restrictions for API calls. One or more API stages, which stand for the deployed versions of the API, can be connected to the consumption plan.
- The API owner creates API keys and associates them with the usage plan. Each key is associated with a specific set of throttling and quota limits defined in the usage plan.
- A client (such as an application or user) obtains an API key from the API owner and includes it in API requests to authorize access to the API.
- When API Gateway receives an API request, it checks the API key against the associated usage plan to determine whether the request is allowed. If the request exceeds the throttling or quota limits, API Gateway returns an error response to the client, (429) Too many requests.
- API Gateway logs information about API requests, including the API key used and the usage against the throttling and quota limits defined in the associated usage plan. It would be prudent to restrict emitting data to Amazon CloudWatch since there may be sensitive client information, for example, you may just want to keep logs of usage and client id.
API owners can finely restrict and monitor access to their APIs using usage plans, enabling them to make sure that their API consumption stays within set parameters.
In addition, API Gateway offers a selection of logging and monitoring tools to assist API owners in comprehending and analysing API usage patterns.
Taking control of your API
Usage plans can be used to control and monitor access to APIs in API Gateway. Therefore, clients that consume APIs deployed in API Gateway can make use of usage plans to ensure that their API usage is within the limits set by the API owner.
Photo by Joshua Hoehne on Unsplash
For example, the API owner can associate an API key with a usage plan that specifies the throttling and quota limits for the client’s API usage.
The API key is compared to the associated consumption plan by API Gateway when the client submits a request to the API. These keys are then distributed to the clients requiring access to the API.
A client might use an AWS SDK or a REST client to make requests to an API deployed in API Gateway. The client can include an API key in the request to authorize access to the API.
The API Gateway sends the client an error message if the request goes over the throttling or quota restrictions. (429) Too many requests
In any other case, API Gateway sends the client’s request to the backend service and receives a response from it.
In summary, clients that consume APIs in API Gateway can make use of usage plans to ensure that their API usage is within the limits set by the API owner.
Let’s examine a situation
Photo by Markus Winkler on Unsplash
Consider that you have an Amazon API Gateway that gives users access to local weather data. To prevent abuse and to guarantee that your API is still accessible to all users, you should set a restriction on the number of requests a client may submit to your API.
To do this, you can create a usage plan that limits the number of requests a client can make per day. You can set the rate limit to 1000 requests per day, for example. The first factor to consider is the usage patterns of the API, including the number of requests typically made per user or application, and the peak usage times. This information can help determine the optimal rate limit that balances the needs of the users with the API provider’s resources. The rate limit should be set at a level that can be supported by the available resources to avoid service interruptions or degradation.
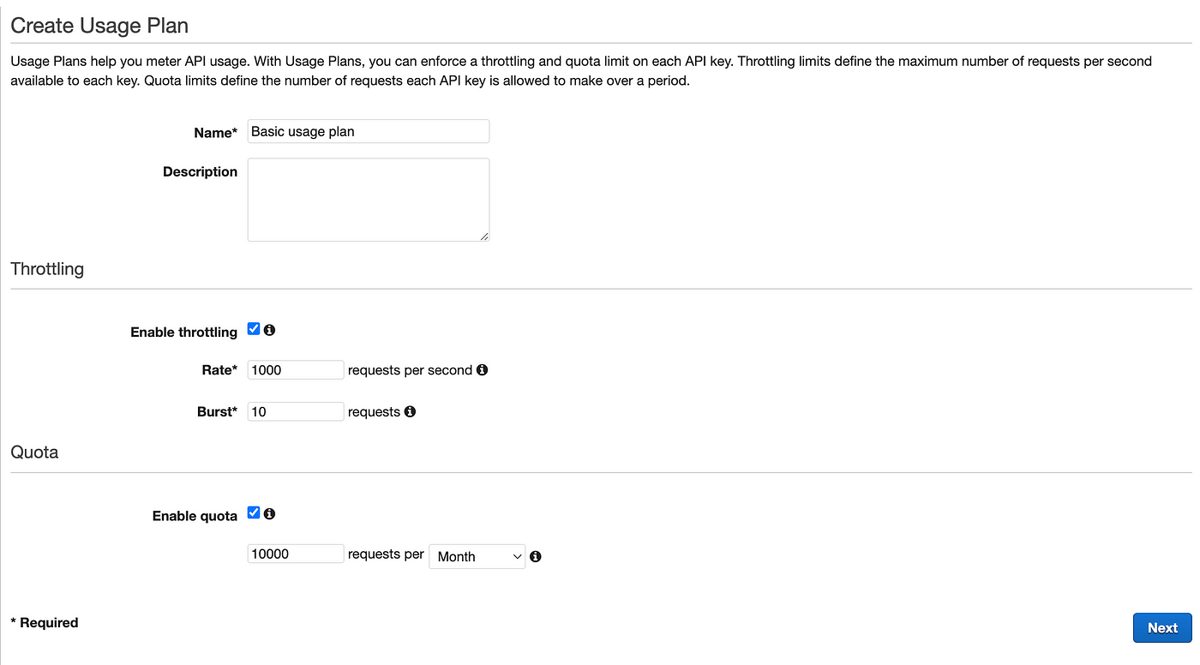
Usage plan on the console
Specify a quota limit in addition to the rate limit. The quota limit specifies the most requests a client may submit in a given period of time. You may, for instance, set a monthly quota cap of 10,000 requests.
You can also specify other parameters in your usage plan, such as the method, resource path, and stage. For example, you can specify that the rate and quota limits apply only to the GET method on the /weather/city resource path in the production stage. Once you have created your usage plan, you can associate it with one or more API keys or IAM roles to control access to your API.
Using the SDK in a Lambda Function
To retrieve usage data for Amazon API Gateway, you can use the getUsage
and getUsagePlan
API calls in the AWS SDK or the API Gateway Management API.
const AWS = require('aws-sdk');const apigateway = new AWS.APIGateway();const params = { usagePlanId: 'my-usage-plan-id', startDate: '2023-01-01T00:00:00Z', endDate: '2023-01-31T23:59:59Z',};apigateway.getUsage(params, function(err, data) { if (err) console.log(err, err.stack); else console.log(data);});
The getUsage
method is called on the AWS.APIGateway
service client to retrieve usage data for the usage plan with the ID "my-usage-plan-id" for the month of January 2023. The API Gateway Management API also provides additional APIs for retrieving usage data, such as getUsagePlan
, getUsagePlanKey
, and getUsageKeys
.
The response from the getUsage
API call will include usage data for the specified time range, such as the number of requests made by each API key associated with the usage plan. You can customise the parameters passed to the getUsage
method to retrieve usage data that meets your specific requirements. The example shows how to request time range parameters:
const params = { usagePlanId: 'my-usage-plan-id', startDate: '2023-01-01T00:00:00Z', endDate: '2023-01-31T23:59:59Z',};
This is what a example response may look like when requesting the getUsage method:
{ "items": [ { "usagePlanId": "abcdefghij", "startDate": "2022-01-01T00:00:00Z", "endDate": "2022-01-02T00:00:00Z", "position": null, "values": { "/example/path": 100, "/another/path": 50 } } ]}
https://docs.aws.amazon.com/apigateway/latest/api/API_GetUsage.html
const AWS = require('aws-sdk');const apigateway = new AWS.APIGateway();const params = { usagePlanId: 'my-usage-plan-id',};apigateway.getUsagePlan(params, function(err, data) { if (err) console.log(err, err.stack); else console.log(data);});
The getUsagePlan
method is called on the AWS.APIGateway
service client to retrieve information about the usage plan with the ID "my-usage-plan-id". The response from the getUsagePlan
API call will include information about the usage plan, such as its name, description, throttling settings, and associated API stages and API keys.
Example response of how it may look when sending a request:
{ "id": "abcdefghij", "name": "exampleUsagePlan", "description": "An example usage plan", "apiStages": [ { "apiId": "1234567890", "stage": "prod", "throttle": { "burstLimit": 100, "rateLimit": 50.0 } } ], "throttle": { "burstLimit": 1000, "rateLimit": 500.0 }, "quota": { "limit": 10000, "offset": 0, "period": "MONTH" }}
You can customize the parameters passed to the getUsagePlan
method to retrieve information about a specific usage plan that meets your specific requirements.
AWS Step Functions to monitor usage
Occasionally, you might wish to keep an eye on how clients are using the API, but when there are lots of clients involved, things become challenging.
In order to gather consumption information for each client, Step Functions can connect all necessary function calls
Note: Here you are making the assumption that there is a database that is populated with items, and these items consist of the API key IDs for the clients. The reason for this is that you need to run a query loop in parallel for each client's key IDs.
Here’s an example of how you can create a state machine (Step Function) that uses the API Gateway Usage Plan SDK to generate a report of consumption by API Key ID. It will start off with a query of keys for each client and run in parallel searching for each client’s usage and usage plans. In the end, there is a Lambda function that will gather information to compute different usage information for your needs.
Some examples of why you might want a lambda at the end:
- Generate reports for client usage
- Create custom logs for cloud watch
- Use computed data to enrich usage monitoring
The list is endless, depending on your needs and strategies.
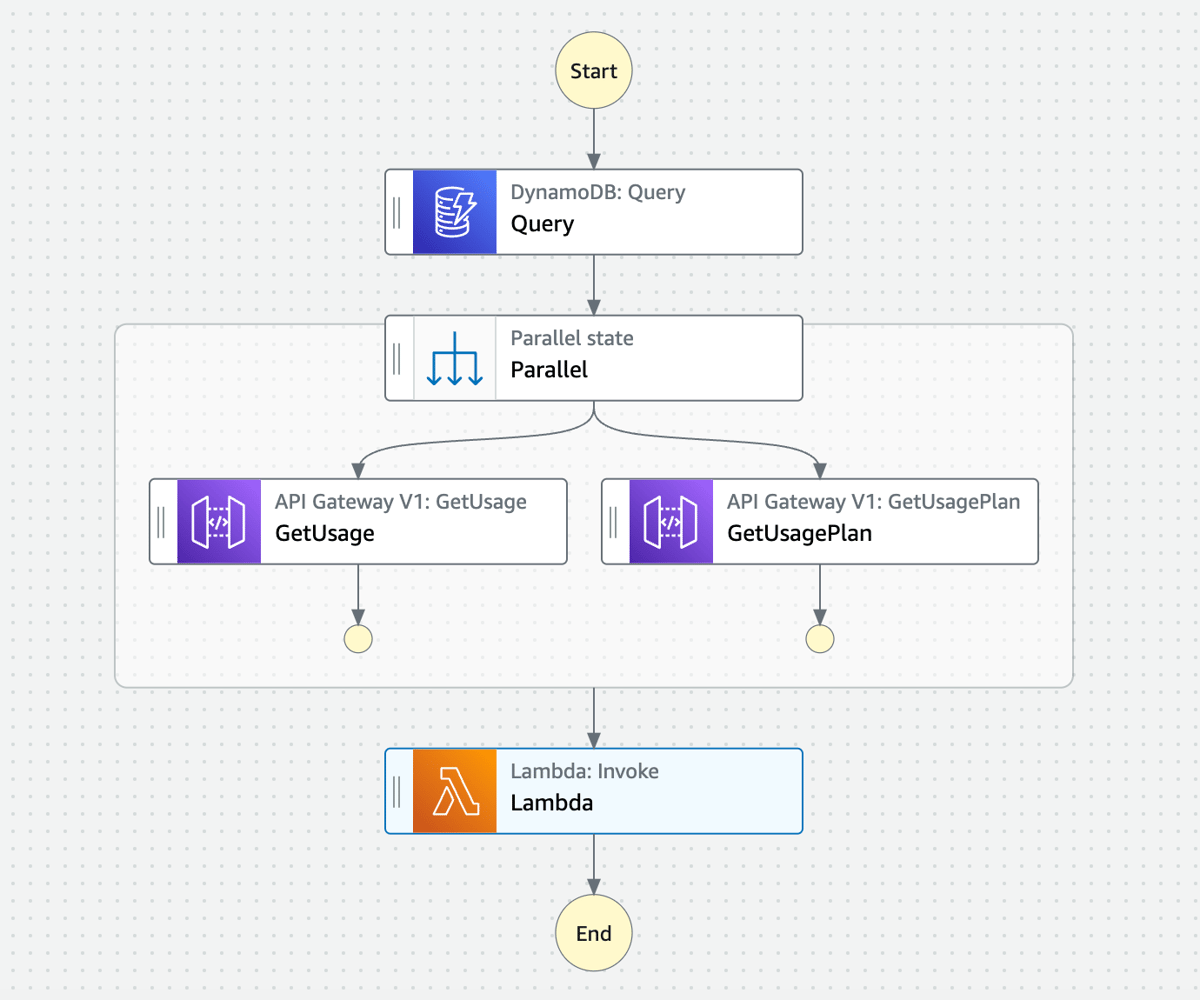
Step Function for usage plans
The aforementioned architecture demonstrates how to develop a Step Function to monitor multiple clients' consumption and usage. It is a useful tactic that enables meaningful monitoring of clients’ daily API activity and thus gives you transparency and the capability to make decisions about your API.
API providers can set quotas that strike the ideal balance between availability, performance, and client usage needs by adopting a data-driven and user-centered strategy.
Adapting your strategy
Once you have a strategy in place, whether it's a simple lambda service or a complex Step Function you can adapt it to integrate other external or monitoring extensions.
To maximise the benefits of the usage monitoring strategy. Great techniques include:
- Alerting to communication platforms like Teams, slack, etc
- Email notifications to replenish quotas
- Query the logs on monitoring platforms
To conclude…
In summary, AWS usage plans are needed to provide API owners with control, visibility, and security over their APIs while also enabling monetization opportunities. By using usage plans, API owners can ensure that their APIs are used in a way that is consistent with their business needs while also providing a good user experience for their clients. These are just some of the ways in which you can create a highly visible and transparent system for monitoring your clients' usage.
AWSAPIApi GatewayLambda Function
Full Article on: https://medium.com/lego-engineering/optimizing-amazon-api-gateway-use-strategies-for-effective-usage-plans-4a72744a16b7