Introduction
The actor model is a programming model in which each actor is a lightweight, concurrent, immutable object that encapsulates a piece of state and corresponding behavior. Actors communicate exclusively with each other using asynchronous messages.
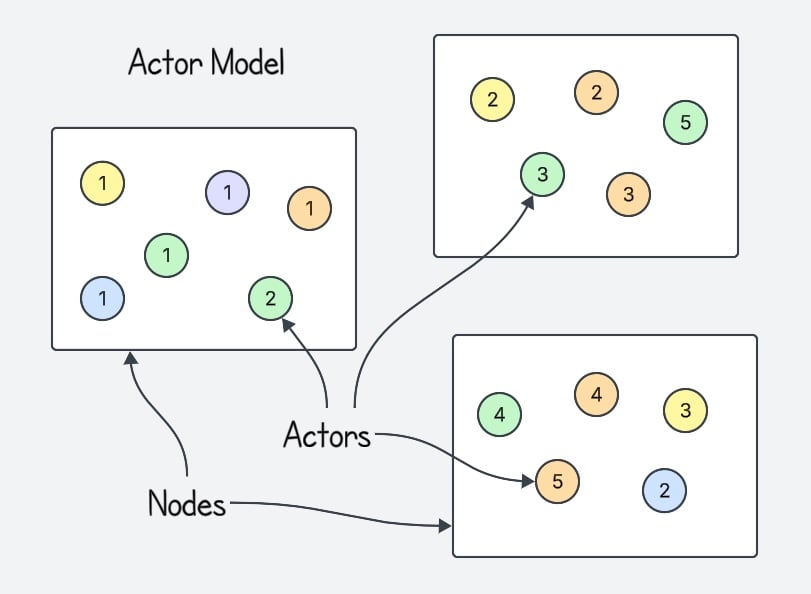
Why You Should Care About the Actor Model
In today's landscape of distributed systems, cloud computing, and real-time applications, developers face mounting challenges: managing concurrency, ensuring fault tolerance, and scaling applications across multiple cores or servers. Traditional programming models that rely on shared state and locks quickly become bottlenecks, leading to race conditions, deadlocks, and code that's difficult to reason about.
The actor model offers an elegant solution to these complex problems. By providing a simple yet powerful abstraction, it allows developers to build systems that naturally handle concurrency, distribution, and failures without the mental overhead of traditional approaches.
What Problems Can the Actor Model Solve?
- Concurrency Complexity: Eliminates the need for complex locking mechanisms and thread synchronisation.
- Scalability Bottlenecks: Provides a straightforward path to scale from a single core to multiple machines.
- Error Propagation: Contains failures within individual actors, preventing system-wide crashes.
- Distributed Computing Challenges: Offers a unified programming model that works the same on one machine or across a cluster.
- Resource Utilisation: Enables efficient use of computing resources through lightweight actor instances.
Fundamental Concepts
Actors
Actors are the primary units of computation in the actor model. Each actor is self-contained, maintaining its own private state and defining behavior to process incoming messages. Actors do not share memory and can only interact through message passing. This design ensures that the system remains concurrent and avoids race conditions.
Actor References and Addresses
Each actor in a system has a unique address or reference that other actors use to send messages to it. These references serve as capabilities that allow one actor to communicate with another without needing to know its physical location. Actor references can be passed in messages, enabling dynamic communication patterns and flexible system topologies.
Message Passing
Actors communicate by sending immutable messages. Since there is no shared state, actors interact by transmitting messages asynchronously. The recipient actor processes the message in its own execution context, enabling non-blocking, distributed, and scalable computation.
Mailboxes
When an actor receives a message, it doesn't necessarily process it immediately. Instead, messages are queued in the actor's "mailbox" - a message queue that stores incoming messages until the actor is ready to process them. This mailbox mechanism is crucial as it:
- Buffers communication, allowing senders to continue their work without waiting.
- Preserves message order (typically in FIFO order, though priorities can be implemented).
- Helps manage load by queuing messages during high-traffic periods.
Message Processing Guarantees
A key characteristic of the actor model is that each actor processes only one message at a time (sequential processing). This property eliminates the need for locks or synchronization primitives within an actor's logic, greatly simplifying concurrent programming. By handling messages one after another, actors can safely modify their internal state without worrying about race conditions, making concurrent systems much easier to reason about.
Location Transparency
The actor model provides location transparency, meaning that the physical location of an actor (whether it's in the same process, on another core, or on a different machine across a network) is abstracted away from the developer. Actors communicate with each other using the same messaging patterns regardless of their physical location. This principle enables systems to be designed without concern for deployment topology and allows for flexible scaling and migration strategies as requirements evolve.
Actor Lifecycle
Actors follow a well-defined lifecycle:
- Creation: Actors are instantiated by existing actors or a parent actor system.
- Processing: An actor receives and processes messages sequentially.
- Modification: Actors can update their internal state in response to messages but cannot modify the state of other actors directly.
- Termination: Actors can stop processing messages and be removed from the system when no longer needed.
Benefits of the Actor Model
- Concurrency: Since actors do not share memory, multiple actors can process messages independently, making it easy to achieve parallel execution.
- Scalability: The asynchronous nature of message passing allows actor-based systems to scale efficiently across multiple cores and distributed networks.
- Fault Tolerance: Actor hierarchies support supervision strategies, where parent actors can monitor and restart failing child actors, making the system resilient to failures.
- Encapsulation: By keeping state private within actors, the model enforces strong encapsulation, reducing unintended side effects.
Comparison with Other Concurrency Models
Understanding how the actor model compares to other approaches helps in selecting the right tool for specific concurrency challenges:
- Traditional Threading with Locks: While familiar to many programmers, this approach often leads to deadlocks, race conditions, and difficulty in reasoning about program behavior. Actors eliminate the need for explicit locking by encapsulating state and using message passing.
- Communicating Sequential Processes (CSP): Models like Go's goroutines and channels also use message passing but typically with synchronous communication. Actors use asynchronous messaging, which can offer better scalability in distributed systems but may introduce more complexity in ensuring message ordering.
- Software Transactional Memory (STM): STM provides atomic, consistent operations on shared memory through transactions. While powerful for specific use cases, STM can suffer from contention under high load. Actors avoid this by eliminating shared state altogether.
- Reactive Streams/Functional Reactive Programming: These focus on data flow and propagation of changes, often using observer patterns. Actors complement these approaches well, particularly when state management and supervision are required alongside reactive flows.
Use Cases
- Distributed Systems: The actor model naturally maps to distributed architectures, allowing systems to scale dynamically.
- Real-Time Systems: Messaging-driven designs are well suited for real-time applications, such as financial trading platforms and chat applications.
- IoT and Microservices: The model provides a foundation for event-driven, microservices architectures, ensuring loose coupling and responsiveness.
Implementations
Several frameworks and programming languages support the actor model:
- Apache Pekko (Scala/Java): Forked from Akka after the licensing model of Akka changed. Provides an open-source toolkit for building actor-based applications on the JVM.
- Orleans (.NET): A virtual actor framework designed for scalable cloud-based applications.
- Erlang/OTP: Erlang's built-in actor-based concurrency model is used in highly reliable systems.
- Ray (Python): A framework for distributed AI and machine learning workloads using an actor-based approach.
Conclusion
The actor model is a powerful abstraction for building concurrent, distributed, and fault-tolerant systems. By leveraging message passing and encapsulation, it simplifies the complexities of shared-state concurrency and enables scalable software architectures. Its adoption across various domains highlights its versatility and effectiveness in modern computing.