In today's rapidly evolving technological landscape, traditional perimeter-based security models are becoming increasingly obsolete. With the rise of cloud computing, microservices architectures, and remote work, organizations need a more robust and adaptable security approach. Zero Trust Architecture (ZTA) has emerged as a compelling framework that addresses these modern challenges.
Understanding Zero Trust: Beyond the Buzzword
Zero Trust isn't merely a product or technology—it's a strategic approach to security that challenges the traditional "trust but verify" model. The core principle is simple yet powerful: "never trust, always verify." Every request, regardless of its origin, must be authenticated and authorized before access is granted.
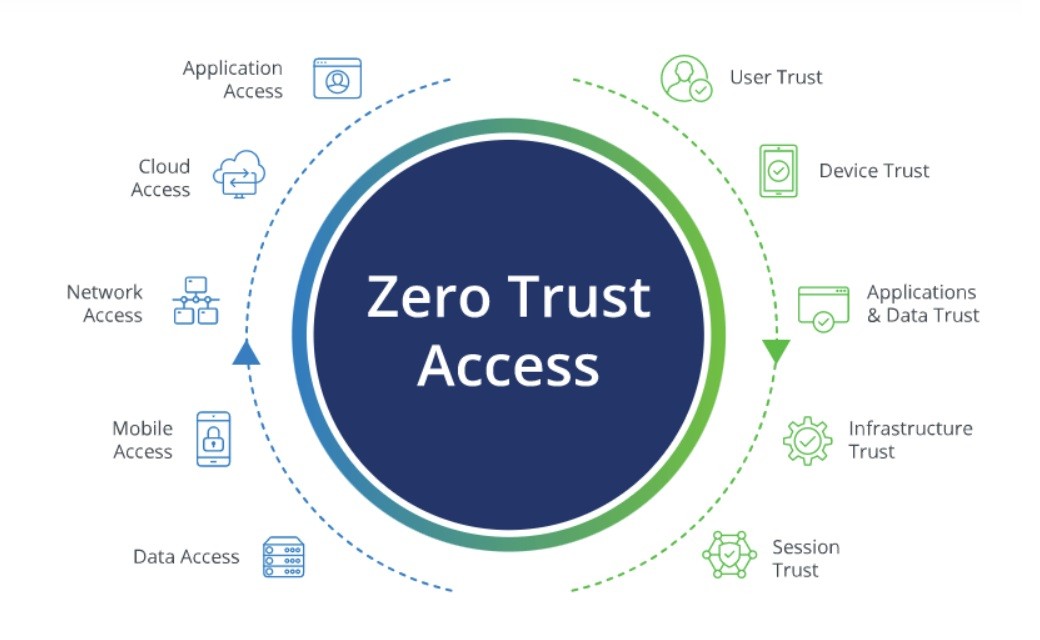
Core Principles of Zero Trust
Identity-Centric Security
In modern cybersecurity, identity has emerged as the cornerstone of secure systems. Unlike traditional security approaches that rely on a well-defined network perimeter, today’s environments demand that every user, device, and application must have a verifiable identity. This ensures that only authenticated and authorized entities can access sensitive systems or data. With the erosion of the conventional perimeter due to cloud adoption, remote work, and mobile devices, identity becomes the new perimeter, offering a more dynamic and scalable way to enforce security policies. Continuous authentication and authorization further strengthen this model by re-evaluating trust in real-time. Instead of relying solely on one-time validation, continuous verification ensures that users, devices, or applications remain trustworthy throughout their sessions, reducing the likelihood of unauthorized access or malicious activities. This approach emphasizes a proactive stance toward security, making it harder for attackers to exploit stolen credentials or compromised devices.
Least Privilege Access
The principle of least privilege access is a fundamental strategy for minimizing security risks by limiting access rights to the minimum necessary for performing specific tasks. Every user, device, or system is assigned only the permissions required to carry out their role—nothing more. This drastically reduces the attack surface, as attackers cannot exploit excessive or unnecessary permissions. Moreover, access rights are not static; they are continuously evaluated and adjusted based on the user’s role, behavior, or changes in their responsibilities. This dynamic model ensures that access aligns with current needs and mitigates risks stemming from outdated or unnecessary privileges. A critical enhancement of least privilege access is the incorporation of time-bound access controls, where permissions are granted only for a specific duration. For example, a user might receive access to sensitive data for a single project or a predefined time window, after which the access is automatically revoked. This ensures that access is temporary and purpose-specific, further reducing opportunities for misuse or exploitation. By implementing least privilege access, organizations can effectively limit insider threats and reduce potential vulnerabilities.
Microsegmentation
Microsegmentation is a sophisticated security strategy that involves dividing a network into smaller, manageable zones or segments, each governed by its own set of access controls. Unlike traditional network segmentation, which often separates networks at a high level, microsegmentation creates granular boundaries within the network, ensuring that sensitive data, systems, and workloads are isolated from one another. Each zone enforces strict access controls, meaning only authorized users or systems can interact with specific resources within that zone. This containment strategy plays a pivotal role in mitigating threats by restricting lateral movement, which is the ability of attackers to move sideways across a network after breaching a single point. For example, even if an attacker compromises one zone, they are effectively trapped and unable to access other parts of the network. Microsegmentation not only enhances security but also simplifies compliance efforts, as specific zones can be designed to meet regulatory requirements. By implementing microsegmentation, organizations can create a highly resilient and controlled network environment, reducing the blast radius of potential breaches while maintaining operational flexibility.
Identity-Centric Security, Least Privilege Access, and Microsegmentation form the foundation of a modern, zero-trust security framework. Together, they address the challenges of today’s dynamic and highly interconnected environments, providing a layered and proactive approach to cybersecurity.
Technical Implementation Strategies
1. Identity and Access Management (IAM)
# Example of implementing JWT-based authentication
from jwt import encode, decode
from datetime import datetime, timedelta
def generate_access_token(user_id: str, roles: list) -> str:
payload = {
'user_id': user_id,
'roles': roles,
'exp': datetime.utcnow() + timedelta(minutes=15),
'iat': datetime.utcnow()
}
return encode(payload, SECRET_KEY, algorithm='HS256')
def verify_access(token: str) -> dict:
try:
payload = decode(token, SECRET_KEY, algorithms=['HS256'])
return {'valid': True, 'payload': payload}
except Exception as e:
return {'valid': False, 'error': str(e)}
2. Network Segmentation
Modern network segmentation goes beyond traditional VLANs, implementing service mesh architectures:
# Example Istio Virtual Service configuration
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: payment-service
spec:
hosts:
- payment.example.com
http:
- match:
- headers:
x-user-role:
exact: payment-processor
route:
- destination:
host: payment-service
subset: v1
3. Continuous Monitoring and Analytics
Implementing real-time security monitoring requires sophisticated logging and analysis:
# Example of implementing security event monitoring
class SecurityEventMonitor:
def __init__(self):
self.anomaly_threshold = 0.95
self.ml_model = load_anomaly_detection_model()
def process_event(self, event: dict) -> bool:
# Extract features
features = self.extract_features(event)
# Calculate anomaly score
anomaly_score = self.ml_model.predict_proba(features)
if anomaly_score > self.anomaly_threshold:
self.trigger_alert(event, anomaly_score)
return True
return False
Best Practices for Implementation
1. Gradual Rollout
Start with a pilot program focusing on:
- High-value assets
- New applications
- Non-critical systems for testing
- Specific user groups
2. Infrastructure as Code (IaC)
Security configurations should be version-controlled and automated:
# Example Terraform configuration for AWS security groups
resource "aws_security_group" "app_sg" {
name = "application-security-group"
description = "Security group for application servers"
vpc_id = aws_vpc.main.id
ingress {
from_port = 443
to_port = 443
protocol = "tcp"
cidr_blocks = ["10.0.0.0/8"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
tags = {
Environment = "production"
ManagedBy = "terraform"
}
}
3. Continuous Security Testing
Implement automated security testing as part of the CI/CD pipeline:
# Example GitHub Actions workflow for security scanning
name: Security Scan
on: [push, pull_request]
jobs:
security_scan:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run SAST
uses: github/codeql-action/analyze@v1
- name: Run Container Scan
uses: aquasecurity/trivy-action@master
with:
scan-type: 'fs'
ignore-unfixed: true
format: 'table'
exit-code: '1'
Common Implementation Challenges
Performance Impact
Performance can be a critical concern when implementing modern security measures. To minimize its impact, it’s essential to adopt strategies that reduce resource load while maintaining robust security. A key solution is to implement caching strategies for frequently accessed resources, such as authentication tokens or user data, which can drastically reduce server overhead and improve response times. Additionally, using efficient authentication mechanisms like JSON Web Tokens (JWT) with appropriately set expiration times ensures secure and lightweight authentication. This approach reduces the need for frequent database lookups, streamlining the authentication process without compromising security. These measures collectively enhance system performance, providing a smoother user experience while maintaining high security standards.
Legacy System Integration
Integrating modern security practices with legacy systems can be challenging but is crucial for maintaining operational continuity. One effective solution is to utilize reverse proxies and API gateways, which act as intermediaries between the legacy system and modern applications. These tools not only provide a secure interface but also enable consistent access control and monitoring. Additionally, adopting gradual migration strategies ensures a smooth transition by phasing out legacy components incrementally while introducing modern replacements. This minimizes disruptions and allows organizations to integrate advanced security features without overhauling the entire system at once. These strategies ensure that legacy systems remain functional while aligning with modern security expectations.
User Experience
Striking a balance between robust security and a seamless user experience is crucial for adoption. One way to achieve this is by implementing Single Sign-On (SSO) where appropriate, enabling users to access multiple systems with a single authentication step, thereby simplifying workflows. Additionally, risk-based authentication dynamically adjusts security measures based on user behavior and context, such as location or device, ensuring that users experience minimal friction during low-risk activities. Lastly, seamless Multi-Factor Authentication (MFA) enhances security without disrupting the user journey by integrating convenient methods like biometrics or push notifications. These strategies collectively improve user satisfaction while maintaining stringent security controls.
Performance Impact, Legacy System Integration, and User Experience with targeted solutions, organizations can achieve a secure environment without sacrificing functionality, compatibility, or usability.
Measuring Success
Security Metrics
Security metrics provide critical insights into the effectiveness of an organization’s security measures and response capabilities. Key metrics include:
• Mean Time to Detect (MTTD): This measures the average time it takes to identify a security incident. A lower MTTD indicates that the organization is efficient in spotting threats quickly.
• Mean Time to Respond (MTTR): This tracks the average time it takes to respond to and resolve a security incident after detection. Reducing MTTR is essential for minimizing damage caused by breaches or attacks.
• Number of Security Incidents: Tracking the frequency of security incidents helps assess the overall security posture and identify patterns or vulnerabilities that require attention.
• Failed Authentication Attempts: Monitoring failed login attempts is critical for detecting potential brute-force attacks or unauthorized access attempts. A sudden spike in failed attempts may indicate a security issue requiring immediate action.
By tracking these metrics, organizations can evaluate their security performance, identify areas for improvement, and respond to threats more effectively.
Operational Metrics
Operational metrics focus on the performance, reliability, and efficiency of systems. They help ensure smooth operations and identify potential bottlenecks or issues. Key metrics include:
• System Latency: This measures the time it takes for a system to process a request. High latency can impact user experience and may signal underlying performance issues.
• Authentication Success Rate: The percentage of successful authentication attempts indicates how effectively the system is functioning. Low success rates may point to technical issues or poor user experience.
• Resource Utilization: Monitoring CPU, memory, and network usage helps ensure resources are efficiently allocated and prevents overloading. Efficient resource utilization is vital for maintaining system performance.
• Application Errors: Tracking the number and types of application errors helps identify software bugs or misconfigurations that could affect system reliability or security.
Together, these metrics provide a comprehensive view of system performance and user experience, enabling proactive management and optimization. Both security metrics and operational metrics are essential for maintaining a secure, efficient, and reliable environment, helping organizations align security practices with performance goals.
Conclusion
Zero Trust Architecture represents a fundamental shift in how we approach security. While implementation challenges exist, the benefits of increased security posture, improved visibility, and reduced attack surface make it a worthwhile investment for modern organizations.
Success in implementing ZTA requires a balanced approach that considers security, performance, and user experience. By following the strategies and best practices outlined in this article, organizations can build robust security systems that are ready for the challenges of the cloud-native era.