The useCallback Promise
In the dynamic world of web development, React has emerged as a transformative player, reshaping how we think about and interact with the digital realm. One of React's game-changing features has been the introduction of hooks, essentially altering the DNA of component design. Among these, useCallback
is a shining star frequently spotlighted in conversations on performance optimization. But does it always live up to the hype? Dive in as we dissect its intricacies.
Expectations vs. Reality
React’s hook system has fundamentally shifted paradigms in component design. Hooks like useState
and useEffect
are now household names for any React developer, but useCallback
holds a special place when discussing performance optimization. However, embracing it without adequate understanding can paradoxically derail performance.
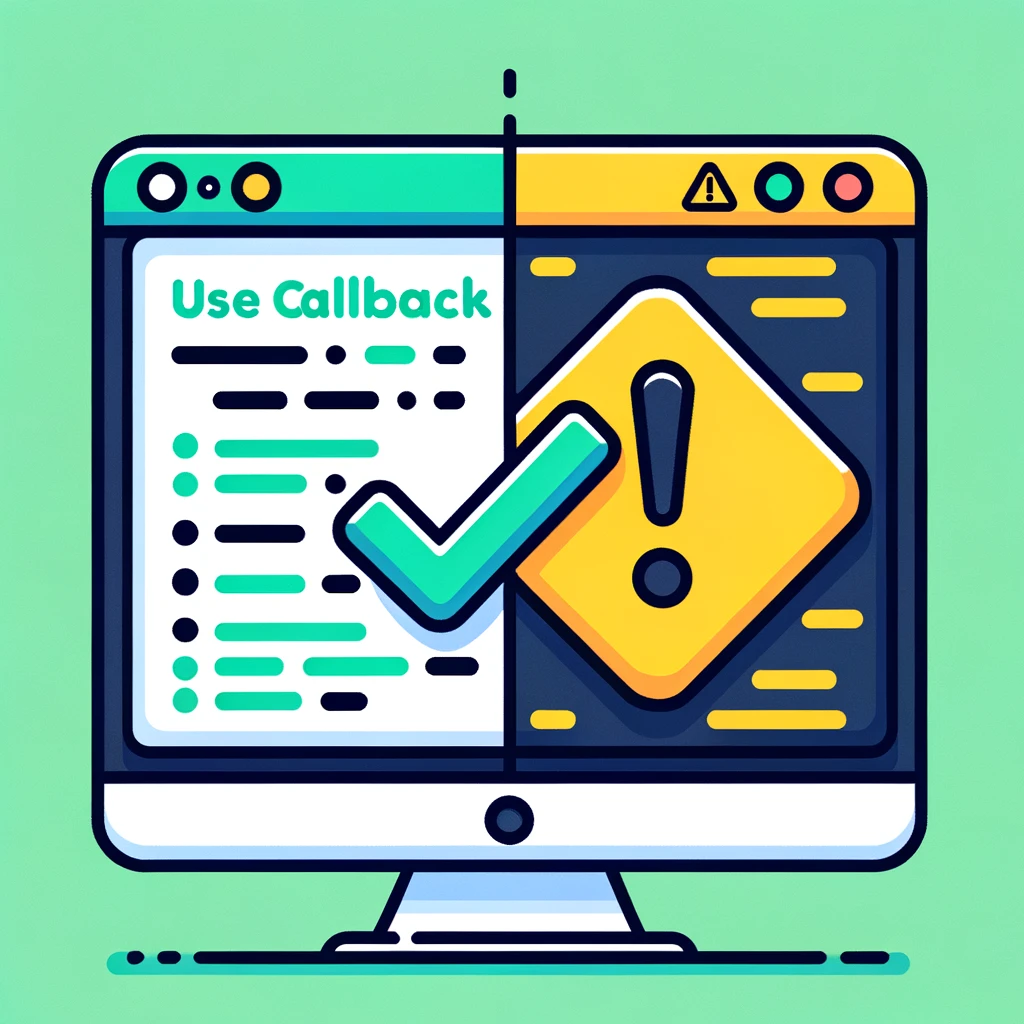
Consider the following example:
function TodoItem({ todo }) {
const onEdit = useCallback(() => {
// Do something with the todo
}, [todo]);
return (
<row>
<text>{todo.text}</text>
<button onclick="{onEdit}">Edit</button>
</row>
);
}
At a glance, integrating the useCallback
hook seems to be a proactive measure for enhancing component efficiency. A closer inspection reveals the following actions during every render:
- Invokes the
useCallback
hook. - Produces a new lambda function, passing it to the hook.
- Constructs a dependency array, feeding it to the hook.
- Internally,
useCallback
assesses dependencies for alterations, subsequently returning the apt function. - Returns the JSX.
Contrast this with the non-useCallback variant:
function TodoItem({ todo }) {
const onEdit = () => {
// Do something with the todo
};
return (
<row>
<text>{todo.text}</text>
<button onclick="{onEdit}">Edit</button>
</row>
);
}
For each render, this simpler version:
- Instantiates a fresh
onEdit
lambda function. - Returns the JSX.
Though the latter seems more straightforward, the essence of useCallback
is anchored in optimization. Nevertheless, it's possible that its introduction inadvertently hampers performance, contingent on the specific use-case.
The Trade-offs of Caching in React
Caching is a double-edged sword. At its core, caching involves storing computations to avoid repeated work. This could mean faster rendering times as unnecessary recalculations are sidestepped. However, there's a price to pay.
Every cached item consumes memory. Over-relying on caching mechanisms like useCallback
might inflate memory usage, slowing down applications or even crashing them in worst-case scenarios. The art lies in discerning when caching brings genuine value and when it becomes a liability.
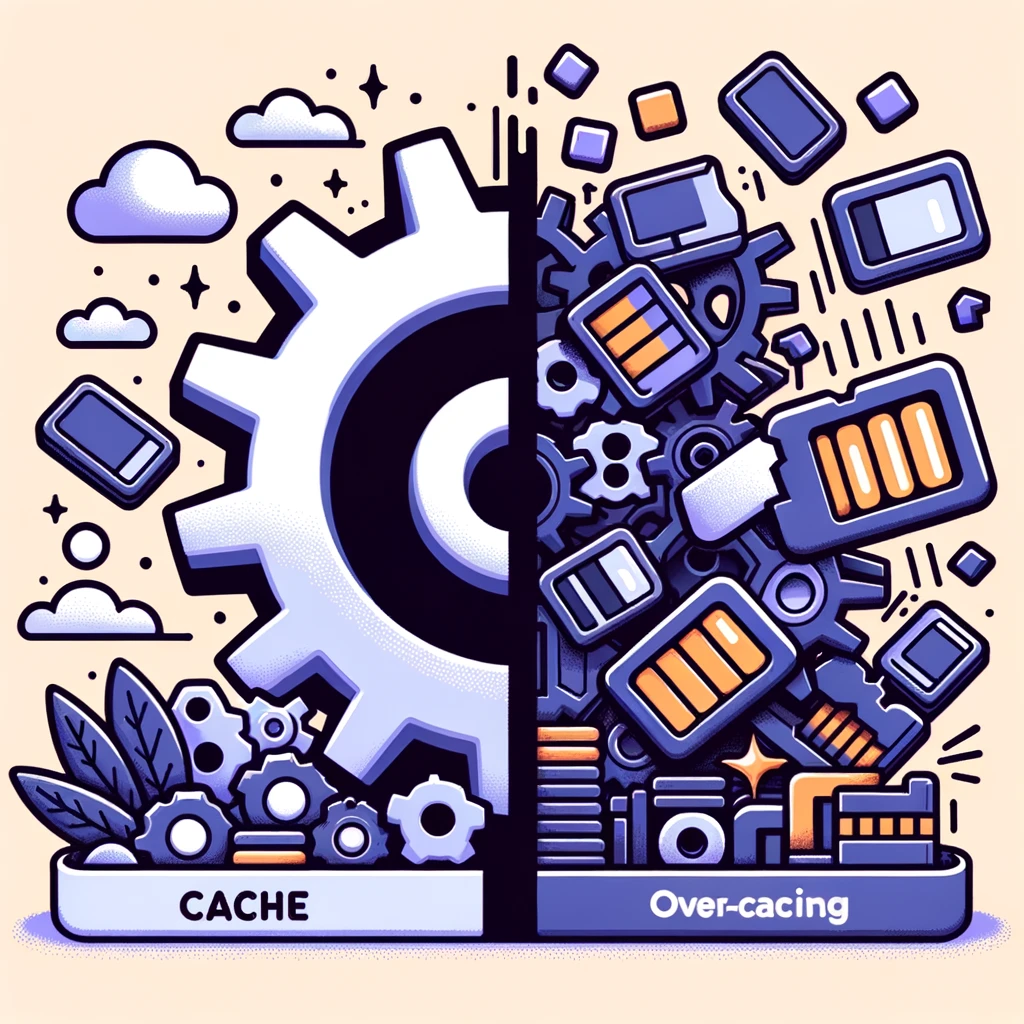
Furthermore, caching introduces complexity. An overlooked expired cache or a misconfigured one can introduce baffling bugs that might be hard to trace and rectify.
Rethinking Callback Placement
Beyond just the useCallback
conundrum lies the broader topic of callback placement. Optimally positioning your callbacks can save more than just performance overhead—it can also make your codebase more manageable and less error-prone.
For instance, if a callback doesn’t depend on any prop or state, why nest it within a component, making it susceptible to needless re-renders? Extracting such functions out, as shown in the example below, can save processing time and enhance code clarity.
const onEdit = (todo) => {
// Do something with the todo
};
function TodoItem({ todo }) {
return (
<row>
<text>{todo.text}</text>
<button =="" onclick="{()"> onEdit(todo)}>Edit</button>
</row>
);
}
The Need for Profiling and Measurement
"Measure twice, cut once." In the vast world of web development, performance cannot be gauged purely by intuition. It's akin to a doctor diagnosing an illness—without the right tests, the diagnosis could be off, leading to ineffective treatments. The React ecosystem provides a suite of tools designed to assist developers in understanding their application's health, both in terms of performance and potential bottlenecks.
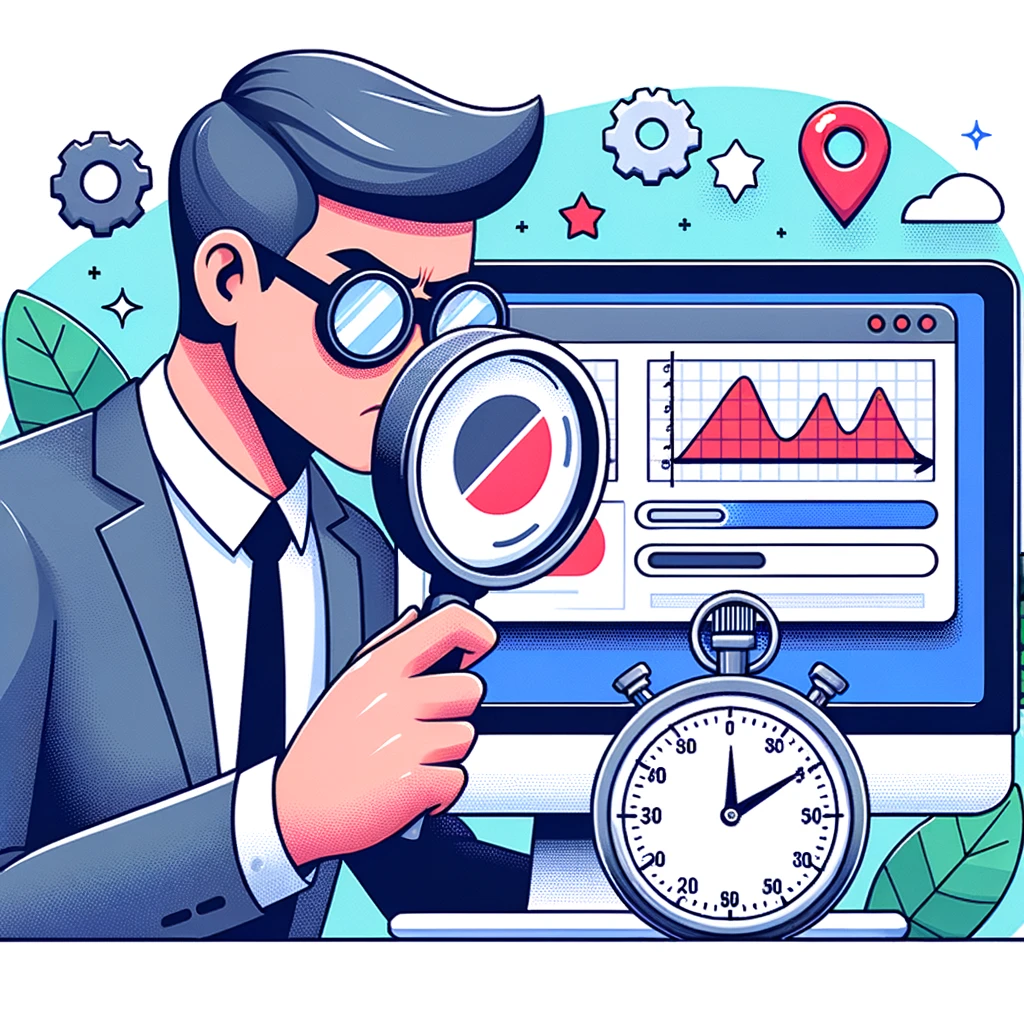
- React DevTools Profiler: One of the most potent tools in a React developer's arsenal, the Profiler tab in React DevTools provides insights into how components render and the costs associated with these renders. For instance, if you've made a change hoping to speed up a particular component, you can use the flamegraph to visually verify the render times before and after your modification. Example: Imagine you've recently incorporated
useCallback
to optimize a list-rendering component. Using the React DevTools Profiler, you can record the rendering process of this component, both with and withoutuseCallback
, comparing the results. You may find that for smaller lists, the difference is negligible, but for larger data sets, the optimization might prove invaluable. Alternatively, you might discover that the useCallback doesn't provide as much benefit as expected, signifying the need for a different optimization strategy. - Chrome's Lighthouse: An automated tool for web page auditing, Lighthouse evaluates web pages across various metrics, including performance, accessibility, and best practices. Lighthouse can generate detailed reports pinpointing areas of inefficiency, especially valuable when considering the broader application context beyond just React-specific rendering. Example: After refactoring a part of your application, you run a Lighthouse audit. To your surprise, even though your React components render faster, the overall page performance score drops. Upon closer inspection, you find that an external third-party script is blocking the main thread. Without Lighthouse's holistic assessment, this crucial performance hit might have gone unnoticed.
- Custom Performance Metrics with the Performance API: Beyond general tools, the web platform offers the Performance API, enabling developers to create custom markers and measures, providing granularity where needed. Example: Let's say you're building a photo gallery with lazy loading images. As users scroll, images are loaded on-the-fly. While you've added this feature to enhance performance, you want to ensure that the loading of images isn't causing noticeable jank or delay in the user experience. By placing markers using the Performance API at the start of the image request and when the image finally loads on the screen, you can measure the actual time taken for this process. If these durations are long, it might indicate the need to further optimize image sizes, consider different formats, or employ other front-end techniques like responsive images or adaptive loading based on network conditions.
For React developers, just as with most types of developers, the journey towards optimisation isn't about making blind adjustments based on trends or hearsay. It's a methodical process, backed by meticulous measurement and profiling. Without these empirical insights, any attempt at optimization might just be shooting in the dark.
Conclusion: Crafting Efficient React Applications
While useCallback
offers a powerful mechanism for specific scenarios, such as maintaining callback reference stability in conjunction with React.memo
, it's essential to discern its appropriate utilization. As you journey through React optimization, always lean on evidence-based decisions, prioritizing empirical evidence over intuition. The harmonization of knowledge, best practices, and continuous learning lays the foundation for efficient React applications.