I recently started doing open-source contributions on GitHub. I have fallen in love with Go as a programming language due to a lot of reasons.
As per Wikipedia, Go is a statically typed, compiled high-level programming language designed at Google by Robert Griesemer, Rob Pike and Ken Thompson. It is syntactically similar to C, but with memory safety, garbage collection, structural typing, and CSP-style concurrency. It is often referred to as Golang because of its former domain name, golang.org but its proper name is GO.
Golang is used to build simple and reliable software with a compromise between performance, control and developer experience. Go is built for software engineering today. It's scalable and compilable on nearly any machine, so you can use it to create a full web app or a tool to clean up incoming data for processing. Go is blazing fast, simple to learn and very easy to rapidly build products. In addition, Go is quickly getting adopted as the most used language across big tech companies including my own.Â
So, I thought to create a simple, elegant and convenient package that can be used by every Go developer to stylize their terminals and enjoy a highly customizable experience. Introducing you to Go-Palette:
Go-Palette provides elegant and convenient style definitions using ANSI colors. It is fully compatible and wraps the fmt library for nice terminal layouts.
The core logic behind Go-palette is using ANSI Colors.
What are ANSI colors?
As per Wikipedia, ANSI escape sequences are standard for in-band signaling to control cursor location, color, font styling, and other options on video text terminals and terminal emulators. Certain sequences of bytes, most starting with an ASCII escape character and a bracket character, are embedded into the text. The terminal interprets these sequences as commands, rather than text to display verbatim.
ANSI sequences were introduced in the 1970s to replace vendor-specific sequences and became widespread in the computer equipment market by the early 1980s. They are used in the development, scientific, commercial text-based applications as well as bulletin board systems to offer standardized functionality.
Although hardware text terminals have become increasingly rare in the 21st century, the relevance of the ANSI standard persists because a great majority of terminal emulators and command consoles interpret at least a portion of the ANSI standard.
An example written using the Go-palette package
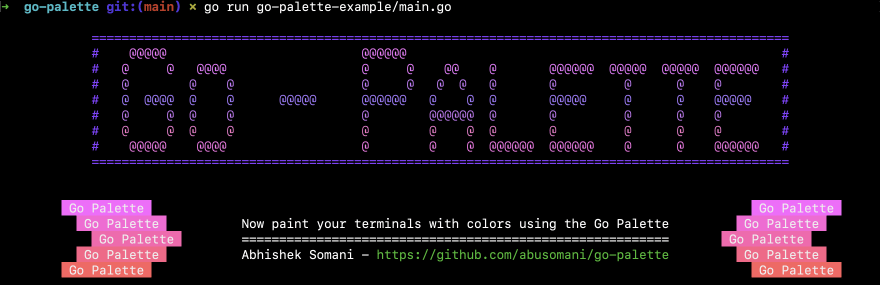
Supported Colors & Formats
Standard colors

The following colors are supported to be used by their names for both foreground and background as shown in the Example using color names
Color Name | Color Code |
---|---|
Black | 0 |
Red | 1 |
Green | 2 |
Yellow | 3 |
Blue | 4 |
Magenta | 5 |
Cyan | 6 |
White | 7 |
BrightBlack | 8 |
BrightRed | 9 |
BrightGreen | 10 |
BrightYellow | 11 |
BrightBlue | 12 |
BrightMagenta | 13 |
BrightCyan | 14 |
BrightWhite | 15 |
Standard colors used as foreground as well as background
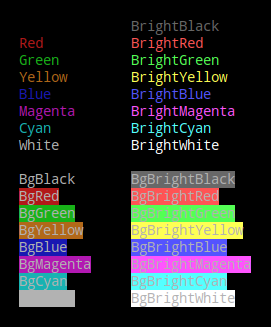
Supported Foreground Palette
The following palette is supported as foreground/text colors. The numbers represent the color codes that can be used as shown in the Example using color codes.
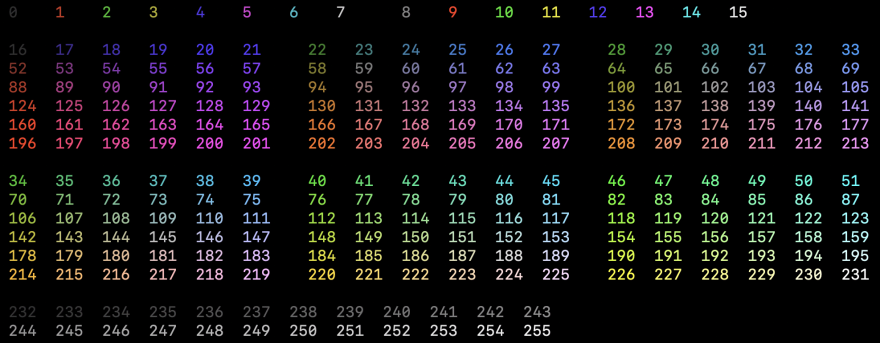
Supported Background Palette
The following palette is supported as background colors. The numbers represent the color codes that can be used as shown in the Example using color codes.
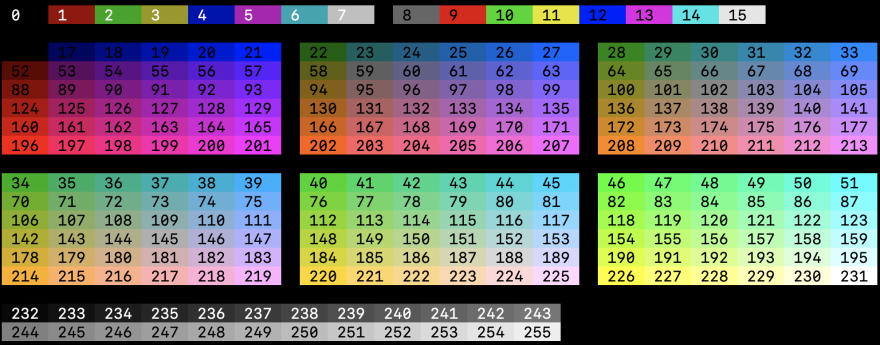
Supported Text Formats
The following text formats are supported.
- Reset
- Bold
- Dim
- Italic
- Underline
- SlowBlink
- Hidden
- Strikethrough

Installation
go get <a href="http://github.com/abusomani/go-palette"><span data-auto-link="true" data-href="http://github.com/abusomani/go-palette">github.com/abusomani/go-palette</span></a>
Usage
After installing the go-palette
package, we start using it the following way.
Import
A very useful feature of Go’s import statement is aliases. A common use case for import aliases is to provide a shorter alternative to a library’s package name.
In this example, we save ourselves having to type palette
every time we want to call one of the library’s functions, we just use pal
instead.
import (
pal "github.com/abusomani/go-palette/palette"
)
Example using Color names
package main
import (
pal "github.com/abusomani/go-palette/palette"
)
func main() {
p := pal.New()
p.Println("This text is going to be in default color.")
p.SetOptions(pal.WithBackground(pal.Color(pal.BrightYellow)), pal.WithForeground(pal.Black))
p.Println("This text is going to be in black color with a yellow background.")
}
Output

Example using Color codes
package main
import (
pal "github.com/abusomani/go-palette/palette"
)
func main() {
p := pal.New()
p.Println("This text is going to be in default color.")
// We can use color codes from the palette to set as foreground and background colors
p.SetOptions(pal.WithBackground(pal.Color(11)), pal.WithForeground(0))
p.Println("This text is going to be in black color with a yellow background.")
}
Output

Example using Special effects
package main
import (
pal "github.com/abusomani/go-palette/palette"
)
func main() {
p := pal.New(pal.WithSpecialEffects([]pal.Special{pal.Bold}))
p.Println("Bold")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.Dim}))
p.Println("Dim")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.Italic}))
p.Println("Italic")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.Underline}))
p.Println("Underline")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.SlowBlink}))
p.Println("SlowBlink")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.Hidden}))
p.Print("Hidden")
p.SetOptions(pal.WithDefaults())
p.Println("<-Hidden")
p.SetOptions(pal.WithSpecialEffects([]pal.Special{pal.Strikethrough}))
p.Println("Strikethrough")
}
Flush
Flush resets the Palette options with default values and disables the Palette.
package main
import (
pal "github.com/abusomani/go-palette/palette"
)
func main() {
p := pal.New()
p.Println("This text is going to be in default color.")
// We can use color codes from the palette to set as foreground and background colors
p.SetOptions(pal.WithBackground(pal.BrightMagenta), pal.WithForeground(pal.Black))
p.Println("This text is going to be in black color with a bright magenta background.")
p.Flush()
p.Println("This text is going to be in default color.")
}
Output

Limitations
Windows
Go-Palette provides ANSI colors only. Windows does not support ANSI out of the box. To toggle the ANSI color support follow the steps listed in this superuser thread.
Different behaviors in special effects
Go-Palette provides styled support using ANSI Color codes through escape sequences. This varies between different Terminals based on their setting. Refer to the ANSI Escape Codes for more details.
More details
Go Pkg Documentation
Thank YouÂ