Concurrency is a powerful feature of the Go programming language that allows developers to write efficient and scalable programs. Go makes it easy to write concurrent programs by providing several high-level abstractions, such as goroutines, channels, the select statement, mutexes, and wait groups.
Goroutines
package main
import "fmt"
func main() {
go fmt.Println("Hello from goroutine 1")
go fmt.Println("Hello from goroutine 2")
}
Channels
Channels are essentially pipes that connect goroutines, allowing them to communicate and share data. When a goroutine needs to send data to another goroutine, it can do so by sending a value over a channel. Similarly, when a goroutine needs to receive data from another goroutine, it can do so by receiving a value from a channel.
Channels are unbuffered by default, which means that sending or receiving from a channel will cause the goroutine to block until the operation can be completed. This makes it easy to write correct concurrent programs, as channels naturally enforce synchronization between goroutines.
However, Go also supports buffered channels, which allow multiple values to be stored in the channel before the receiver is ready to receive them. This can be useful in some scenarios where a goroutine needs to send multiple values to another goroutine without blocking.
package main
import "fmt"
func main() {
// Create a channel that can be used to send and receive strings.
c := make(chan string)
// Create two goroutines that send messages to the channel.
go func() { c <- "Hello from goroutine 1" }()
go func() { c <- "Hello from goroutine 2" }()
// Read the messages from the channel and print them to the console.
fmt.Println(<-c)
fmt.Println(<-c)
}
This code creates a new channel and a goroutine that sends a value on the channel. The main function receives the value from the channel and prints it to the console. Channels are a powerful tool for coordinating and synchronizing goroutines.
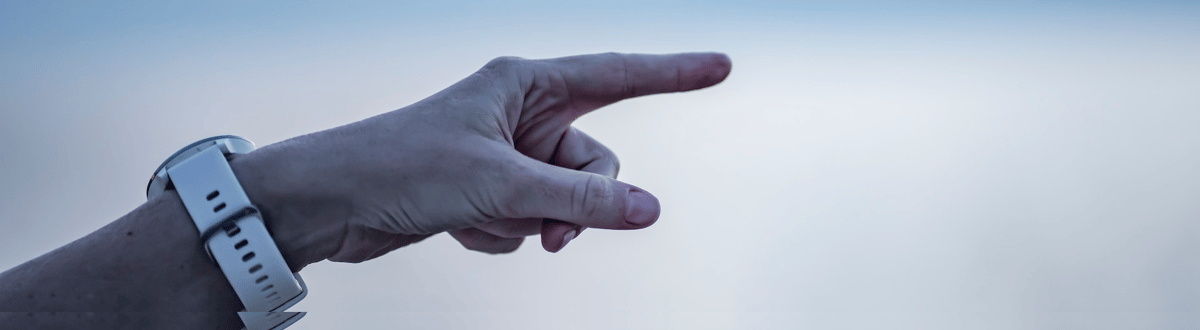
Select
The select statement is a powerful construct that allows a goroutine to wait for multiple channels to become ready. This means that the goroutine can block until one of the channels is ready, then execute a block of code depending on which channel is ready. This makes it possible to write highly efficient and responsive concurrent programs that can handle multiple channels of data without blocking or consuming excessive resources.
The select statement works by allowing a programmer to specify a set of cases, each of which represents a channel that the goroutine is waiting to become ready. When one of the channels becomes ready, the corresponding case is executed, and the goroutine continues to execute the code associated with that case.
The select statement can also be used with a default case, which is executed if none of the other cases are ready. This can be useful for situations where the goroutine needs to perform some other action if none of the channels become ready.
package main
import (
"fmt"
"time"
)
func main() {
// Create two channels: one for sending a signal after 1 second,
// and one for sending a signal after 2 seconds.
c1 := time.After(1 * time.Second)
c2 := time.After(2 * time.Second)
// Use a select statement to wait for one of the channels to become ready.
select {
case <-c1:
fmt.Println("1 second elapsed")
case <-c2:
fmt.Println("2 seconds elapsed")
}
}
Mutex
One of the most common problems that can arise in concurrent programs is race conditions, which occur when multiple goroutines access shared data at the same time, potentially leading to inconsistent or incorrect results. To prevent these kinds of issues, Go provides a mutex type that can be used to protect critical sections of code.
A mutex is a data structure that is used to provide mutual exclusion to a critical section of code. When a goroutine wants to access a critical section, it first acquires the mutex, which prevents any other goroutines from accessing the same section of code. Once the goroutine has finished executing the critical section, it releases the mutex, allowing other goroutines to acquire it and execute the same section of code.
Mutexes in Go are implemented using the sync package, which provides several different types of mutexes, including both mutexes that can be held by only one goroutine at a time (called a "standard" mutex), as well as more complex mutexes that allow for more fine-grained control over access to shared resources.
In addition to providing mutual exclusion to critical sections of code, mutexes can also be used to synchronize access to shared resources. For example, if multiple goroutines need to access a shared data structure, a mutex can be used to ensure that only one goroutine is accessing the data structure at any given time, preventing race conditions and other issues.
package main
import (
"fmt"
"sync"
)
func main() {
// Create a mutex to protect the counter variable.
var mu sync.Mutex
// Create a counter variable and initialize it to 0.
var counter int
// Create 10 goroutines that concurrently increment the counter.
for i := 0; i < 10; i++ {
go func() {
mu.Lock()
defer mu.Unlock()
counter++
}()
}
// Wait for all the goroutines to finish, then print the final value of the counter.
time.Sleep(1 * time.Second)
fmt.Println(counter)
}
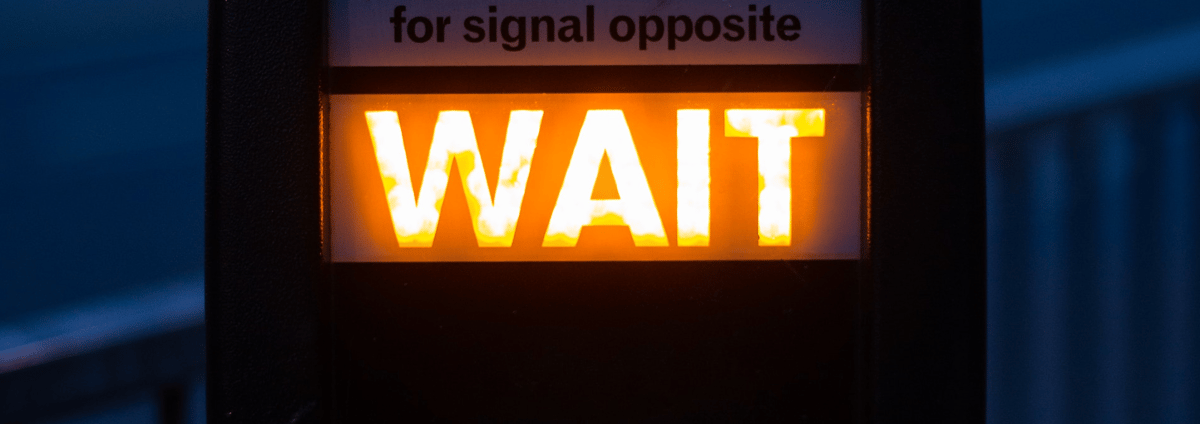
Wait Groups
One of the most important packages for working with concurrency in Go is the sync package, which provides a variety of tools for managing concurrent code.
One of the most useful tools provided by the sync package is WaitGroups, which can be used to coordinate the termination of multiple goroutines. WaitGroups are essentially counters that can be incremented or decremented by goroutines, allowing a program to wait for all the goroutines to finish executing before continuing. This can be especially useful for tasks such as parallel processing or fan-out/fan-in patterns, where a program needs to wait for multiple goroutines to finish before continuing.
Another tool provided by the sync package is Once, which can be used to ensure that a piece of code is only executed once. Once is essentially a type of mutex that allows a piece of code to be executed only once, even if multiple goroutines attempt to execute it simultaneously. This can be useful for initializing global variables or performing other one-time operations.
Finally, the sync package also provides a Pool type, which can be used to manage and reuse a pool of resources. Pools can be used to reduce the overhead of creating and destroying resources, by reusing existing resources instead of creating new ones each time they're needed. This can be especially useful for resources such as network connections or database connections, which can be expensive to create and destroy.
In addition to these tools, the sync package also provides several other types and functions for working with concurrency in Go, such as Mutexes, Cond, and Once. These tools can be used to ensure the correctness and efficiency of concurrent programs, and are essential for writing high-performance and scalable concurrent code in Go. Here's an example of how to use the WaitGroup type from the sync package to wait for a group of goroutines to finish:
package main
import (
"fmt"
"sync"
)
func main() {
// Create a WaitGroup to wait for the goroutines to finish.
var wg sync.WaitGroup
// Launch 10 goroutines that concurrently print messages.
wg.Add(10)
for i := 0; i < 10; i++ {
go func() {
fmt.Println("Hello from goroutine")
wg.Done()
}()
}
// Wait for the goroutines to finish.
wg.Wait()
}